I found out today that it is Restaurant week in DC. I quickly found the official website, and equally quickly found out that it was hard for me to figure out things I wanted to know, like where these restaurants are, and what Yelp thinks of them. The only logical conclusion was to make a website do exactly that. I ended up using my site to find a place to eat for dinner, and it was delicious 🙂
Currently, I’ve overrun my Yelp requests for the day, I’m going to work on getting the Yelp functionality integrated again, ASAP.
Check out the site at r.prometheusx.net, what do you think?
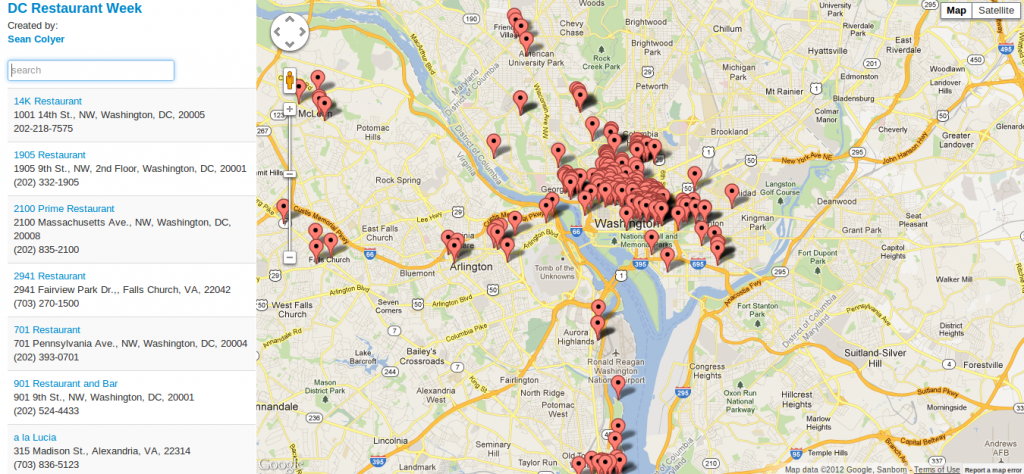
Technical Details
Gathering the information
In order to gather information about all of the restaurants from the official site at first I was a little unsure. Then I realized I could just use jQuery to gather all of the elements and create a json object that I could use directly in this page.
Below is the bulk of my parsing code, I was then able to JSON.stringify an array of these restaurant objects to easily copy the data.
$('.formfont_black b').each(function (){ var parentColumn = $(this).closest('td'); var restaurant = {}; restaurant.name = $(this).html(); restaurant.url = $(this).closest('a').attr('href'); var lineSplit = parentColumn.html().split('<br>'); restaurant.addr = lineSplit[1]; restaurant.phone = lineSplit[2]; } |
What database?
I thought about setting up a quick Rails application for the backend of this, but then realized that there was really limited value in a database since this information is all static, and there’s not that much of it. Therefore, I’ve dumped most of the content directly into the javascript files. If this were a more serious application this could easily be adjusted.
Third Party Integration
Google – I quickly realized that the geocoding API for Google Maps was severely going to throttle my ability to look up restaurants. It limits you to, I believe, ~11 queries per second. Therefore, in order to map all 250 restaurants, I mapped them once and just saved that data into a JSON map, the same way as the restaurant information.
I’ve pasted my hacked together method to get all of the geocoded information. I was then able to JSON.stringify() the result
function geocode_address(map, geocoder, restaurant){ geocoder.geocode( {address:restaurant.address}, function(results, status){ if (status == google.maps.GeocoderStatus.OK) { var marker = new google.maps.Marker({map:map, position:results[0].geometry.location}); google.maps.event.addListener(marker, 'click', function(){ yelpRequest(restaurant, marker); }); good++; goodAddresses[restaurant.address] = results[0].geometry.location; } else { bad++; setTimeout(function(){ geocode_address(map,geocoder,restaurant); }, 100); } }); |